Gathering Usage Data with the Azure Usage API
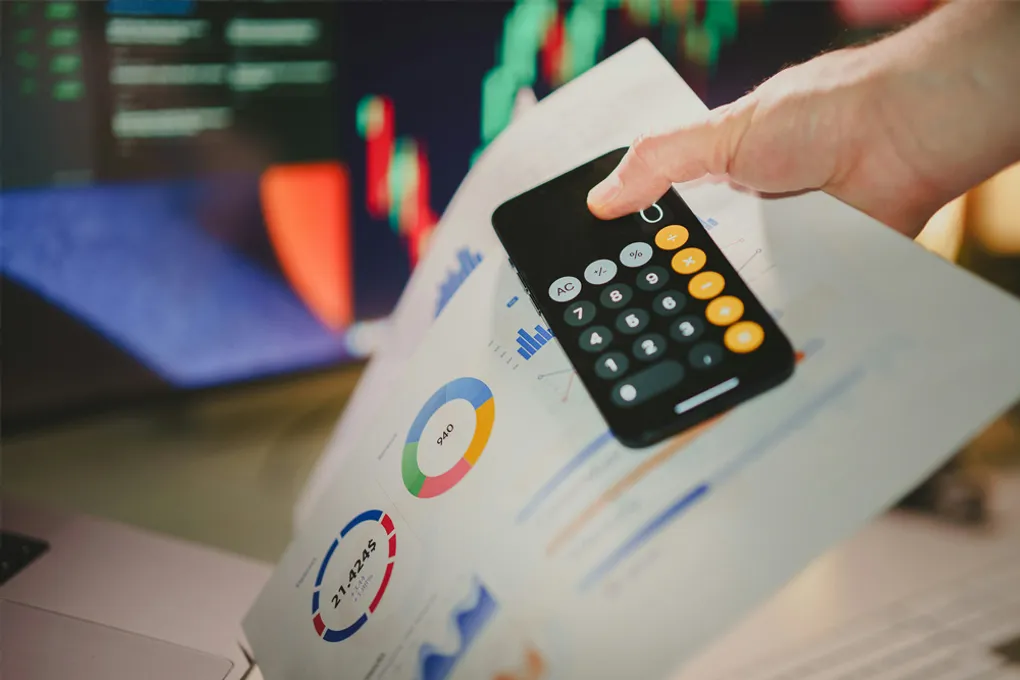
The Azure Rest API is very extensive and can be used to gather a lot of information about your Azure resources. The Azure Rest API allows you to gather usage data, something I had to do recently when trying to find what resource types had been in use over the last 12 months.
Alternative Options
Before we go to far in to the Usage API it is worth mentioning that there are other options for gathering usage data.
If you want to query your Azure account for the current resources the Azure Resource Graph is a great option and you can use KQL to interrogate and aggregate the data. It is worth noting though that it only shows the current state of the resources and does not show any deleted resources, historical information or usage data.
Azure Metics API can be used to get granular usage data for your Azure resources, however it is limited to only look back for around three months and does not contain any cost data.
Accessing the Azure Usage API
There are a number of ways to access the Rest API.
- Raw HTTP request providing the correct authentication.
- Azure CLI to make a REST call
- Azure CLI to call
az consumption usage list
- Azure SDK
The preview az consumption usage list
command has an open issue when using a date range and I also had issue when using it on a pay as you go Azure account. Therefore for this article we will use the Azure CLI to make a REST call. By using the Azure CLI we can authenticate using the current session and avoid having to manage the authentication ourselves.
Code
The following code will query the Azure Usage API for the last 12 months of usage data. The code will loop through the results and save each page of data to a json file, each page provides a link to the next. This will allow us to download all the data and then process it as needed in a later script.
The results provide a lot of different information including the product name, usage quantity, pricing, currency, resource Id, location and tags, all of which can be seen in the billing section of the Azure Portal.
This code I have written is in powershell and it uses the Azure CLI to make the REST call however you could just as easily use the Azure Powershell commands.
# Ensure you are logged in to Azure
$status = (az account show)
if ($status.length -eq 0) {
az login --output none
}
# Set the subscription you want to query
$subscriptionId = "[Your Subscription ID]"
az account set --subscription $subscriptionId
# Query the usage data for 2024 (Note the date must be in the format "yyyy-MM-dd HH:mm:ssZ")
$startDate = "2024-01-01 00:00:00Z"
$endDate = "2025-01-01 00:00:00Z"
$url = "https://management.azure.com/subscriptions/$subscriptionId/providers/Microsoft.Consumption/UsageDetails?api-version=2021-10-01&`$filter=properties/usageStart ge '$startDate' and properties/usageEnd le '$endDate'"
$counter = 1
$nextLink = $url
while ($true) {
$usageDetails = az rest --method GET --url $nextLink --output json
$usageDetails | Out-File -FilePath "usageDetails-$counter.json"
if ($usageDetails | ConvertFrom-Json | Get-Member -Name nextLink) {
$nextLink = $usageDetails | ConvertFrom-Json | Select-Object -ExpandProperty nextLink
}else{
break
}
$counter++
#We don't know how many pages there are so we can't give a percentage complete
Write-Progress -Activity "Fetching usage details" -Status "In Progress $counter Downloaded"
}
Write-Progress -Activity "Fetching usage details" -Status "Completed"
Limitations
Although this gives you a good way to see historical usage data it will only include billable items. This means that if you have a service such as a network security group that is not billed you will not see it in the usage data.
Conclusion
The Azure Usage API is a powerful tool that can be used to gather usage data for your Azure resources. By using the Azure CLI to make the REST call you can easily authenticate and query the API. This can be useful for monitoring your usage, tracking costs, and optimizing your resources. By saving the data to a file you can download all the data and then process it as needed.
Title Photo by Jakub Żerdzicki on Unsplash